Hello there, pals. Hello and thank you for visiting my blog. We're going to simplify the concept of DOM Manipulation today.
I wrote a very comprehensive and simplified explanation of DOM, if you have not read it check it out Read now. don't forget to like, comment and share.
Introduction
Recently I was asked the question, what is the relationship between JavaScript and webpages
. I have asked myself that question some time ago, I was new to JavaScript then and all I have learned in JavaScript was the logical aspect, function, array, etc.
I called JavaScript boring because I thought it was all about console.log and some additions and subtractions.
Never knowing there is an interesting part which is called DOM, and the more interesting part is DOM manipulation.
learning JavaScript DOM manipulation from other resources was a bit straightforward and has a lot of similarities, let's make a difference. let's add fun to it ๐.
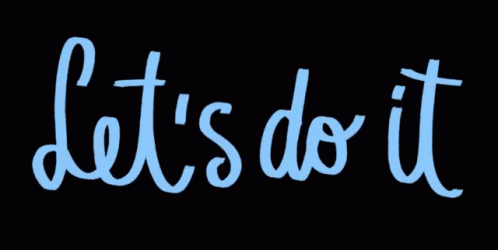
Explanation
What is Manipulation?
Controlling someone or something to your advantage.
Read more
Let us look at it in this manner, controlling components of a webpage and using them at will.
For us to manipulate anything we need to have access to it.
We need access to.
- Document
- Element
Document
A document is refer to has file(HTML file) which houses all the elements, images, links, etc. Represented on the webpage. Accessing the document we will make use of the javascript keyword document
Element
Manipulating an element you have to go through the document. just like driving a car, you have to be in the car
. After entering the document you will have to find (target) the element or group of elements you want to manipulate. Finding an element we have to make use of the keyword getElement then how do you want to query the document?
There are several ways to do this:
- Finding HTML elements by id
- Finding HTML elements by class name
- Finding HTML elements by tag name
- Finding HTML elements by CSS selectors
- Finding HTML elements by HTML object collections
Finding an Element.
We have broken the process of getting an element, let us gather the pieces so it will make sense.
Finding HTML elements by id
This queries the document for an element with the corresponding id, this method will return a single element because HTML "id" is unique and no two elements can have the same ''id". returns undefined if no element is found with the "id"
// <div id="unique"></div>
document.getElementById("unique")
Finding HTML elements by class name
This queries the document for elements with the corresponding class, this method will return HTMLCollection {} read about HTMLCollection , elements because HTML ''class'' is not unique many elements can have the same "class''.returns an empty HTMLCollection {} if no element is found with the "class"
// <div id=" class"></div>
document.getElementsByClassName("my class")
Finding HTML elements by tag name
This queries the document for elements with the corresponding tag, this method will return HTMLCollection of elements you guess it right
because tags can be used multiple times in HTML.returns an empty HTMLCollection {}, if no element is found with the "tag"
// <p >paragraph</p>
document.getElementsByTagName("p")
Finding HTML elements by CSS selectors
An Element object representing the first element in the document that matches the specified set of CSS selectors read about css_selectors, or null is returned if there are no matches it returns a single element.
// For class
document.querySelector(".myclass");
// For Id
document.querySelector("#unique");
// For tag
document.querySelector("p");
querySelectorAll()
The Document method querySelectorAll() returns an HTMLCollection {} representing a list of the document's elements that match the specified group of selectors, more than one query can be sent at a time by using "," to separate them.
document.querySelectorAll("p, #unique, .myclass")
At this point we have gotten the element we want to manipulate
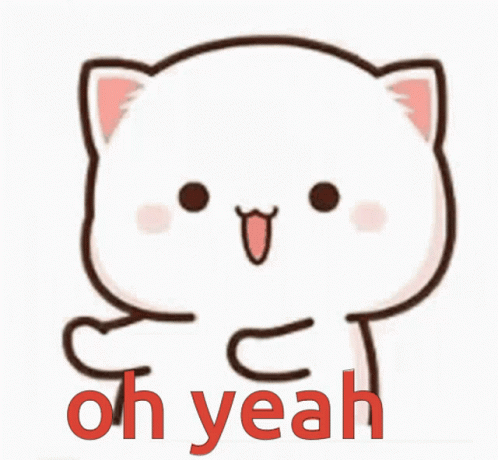
Manipulating an Element.
Manipulating an element is fun, we can change elements into anything we can think of
๐ our creativity is our limitation. Before we move ahead let's list out things we can do using DOM manipulation.
- Create an element
- Create text nodes
- Update text
- Create attribute
- Create element attribute
- Append the new element to DOM
- Style element
- Remove an existing element
- Event listener
- and many more
They are a lot more to list, I should stop here. We will pick them one by one and explain them. Let's get to it
Create an element.
Let's create a new element and store it in a variable so we can make use of it when the variable is been called.
const newElement = document.createElement("div")
We can create any element of our choice, The element will be created and stored in the variable "newElement", so we can make use of the element when we call the variable name.
Create text nodes
The element created is empty and has no text in it. To add a text we have to create a text node. This will be stored in a variable too.
const newText = document.createTextNode("Hi there and greetings!");
At this point, the element and the text are created separately, we have to insert the text into the new element and then insect the new element into the DOM tree, this is when the newly created element and text will be visible on the browser. This is called appending
Appending
- We are to append the created textNode to the newElement.
newElement.appendChild(newText)
Now the text is placed in the newElement has a child node.
newElement.appendChild(newText)
- Appending the newElement to the DOM. At this point, the element is not visible on the webpage. we have to insert the new element into the DOM, for this we need to target an element in the DOM that will be the parent of the new element
//<div id=" parent"></div>
const parentElement = document.getElementById("parent")
parentElement.appendChild(newElement)
Once this is done the element and its text will be on the document DOM tree
This took over 5 to 6 lines of code to archive let me give you an alternative that is simpler and shorter.
Creating an element with a Template literal
Template literals are literals delimited with backtick (`) characters, allowing for multi-line strings, string interpolation with embedded expressions, and special constructs called tagged templates. Read more
// get parent element
const parentElement = document.getElementById("parent")
// create HTML template
const newElement = `<div>Hi there and greetings!</div>`
// insert the HTML template into the parentElement
parentElement.innerHTML = newElement
It has simple has this
For this example, it looks short, but when you will want to add multiple elements to the DOM you will find this helpful.
let's see an example.
let's add an unordered list to the DOM
// get parent element
const parentElement = document.getElementById("parent")
// create HTML template
const newElement = `
<ul>
<li>List item 1</li>
<li>List item 2</li>
<li>List item 3</li>
<li>List item 4</li>
</ul>
`
// insert the HTML template into the parent element
parentElement.innerHTML = newElement
Update text
Javascript DOM text can be updated just by targeting the element you want to change the text and overwriting the previous text.
//<div id="unique"> HTML text</div>
const element= document.getElementById("unique")
element.innerText = "New text from javascript"
Style element
This is getting more fun, styles can also be applied to any HTML element in the DOM with javascript. With this, you can animate any element, hide, slide, popup, etc. Any element of your choice, I said your creativity is your limitation in styling an element with javascript. read more about CSS style property in javascript
//<div id="unique"> HTML text</div>
// Getting the element first
const element= document.getElementById("unique")
// examples for updating styles
element.style.color = "blue";
element.style.backgroundColor = "red";
Create element attribute
The create attribute method creates a new attribute node and returns it. The object created a node implementing the Attribute interface. The DOM does not enforce what sort of attributes can be added to a particular element in this manner. read more
//<div id="unique" data-attribute_name="foo"></div>
const element = document.getElementById("unique");
element.setAttribute('new-attribute, 'attribute-value');
Get an element attribute value
The getAttribute() method of the Element interface returns the value of a specified attribute on the element.
If the given attribute does not exist, the value returned will either be null or "" (the empty string) read more
<div id="unique" data-attribute_name="foo"></div>
var element = document.getElementById("unique");
var attribute_value = element.getAttribute("attribute_name");
Remove an existing element
Removing an existing element from DOM is done in two ways,
- using the remove()
- using the removeChild()
These differences can help you out in some cases if you want to retrieve the child node or in some cases remove it completely
RemoveChild method
Remove child method remove the node, the element removed can be saved in a variable for later use. This method needs a reference to the parent element and then the child to be removed, this is used when you will want to remove an element only within a specific parent element, you target the parent element, and secondly, target the child you want to remove from the DOM.
//<div id=" parent">
//<p id="child">Hi there and greetings!</p>
//</div>
let parentElement = document.getElementById("parent");
let childElement = document.getElementById("child");
parentElement.removeChild(childElement);
Note: As long as a reference is kept on the removed child, it still exists in memory, but is no longer part of the DOM. It can still be reused later in the code. If the return value of removeChild() is not stored, and no other reference is kept, it will be automatically deleted from memory after a short time.
remove() method
The remove method removes the node, the element removed is removed no reference is stored on the memory. This method does not need a reference to the parent element so it is a one way delete from the DOM
const element = document.getElementById("unique");
element.remove();
Event listener
First of all, what is an Event: something that happens or is regarded as happening; an occurrence, especially one of some importance. the outcome, issue, or result of anything
Read more
this is divided into two.
- Registering an event
- Handling an event.
Registering an event
This can be done by adding an event listener has an attribute in the HTML element list of HTML events
<button onClick="myFunction()">Click me</button>
Secondly, an event can be registered in javascript by targeting the element and adding an event lister to it
const element = document.getElementById("unique");
element.addEventListener("click",()=>{
// function
})
Note: The addEventListener() method is the recommended way to register an event listener. The benefits are as follows:
- It allows adding more than one handler for an event. This is particularly useful for libraries, JavaScript modules, or any other kind of code that needs to work well with other libraries or extensions.
- In contrast to using an onXYZ property, it gives you finer-grained control of the phase when the listener is activated (capturing vs. bubbling).
- It works on any event target, not just HTML or SVG elements.
Handling an event
Handling an event is the action that will occur when the listener receives a trigger. for example, a click event will be triggered when they click on the element the event is registered on.
function myFunction(){
// actions
}
Conclusion
We have covered a lot in this article, at this point we have created an element and appended it to the DOM, we have also manipulated existing elements. This is not all about DOM manipulation but put this to practice and follow for more interesting topics.
Congratulations
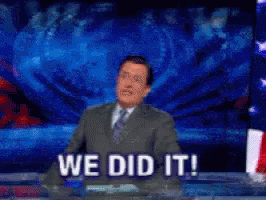
Give a feedback
If you enjoyed and learned something new from this article, Follow me for More web simplified topics and do well to give me feedback by using the reaction emojis.
Let me know how you feel about the explanation in the comment section. Give feedback, corrections, and recommendations.
Much ๐ฅฐ
Let us connect
I will like to connect with any and every reader
Let us connect
victorjosiah19@Twitter
josiah-victor@LinkedIn